In this video lesson, we demonstrate how to create a two-axis tilt meter. The device displays both the pitch and roll on an OLED. In addition to this quantitative display of tilt and roll, it also shows a carpenter’s level type visual, where a circle, or bubble moves to indicate tilt. When the circle is centered on the crosshairs, the device is flat in both axis.
For your convenience, this is the schematic we are using:
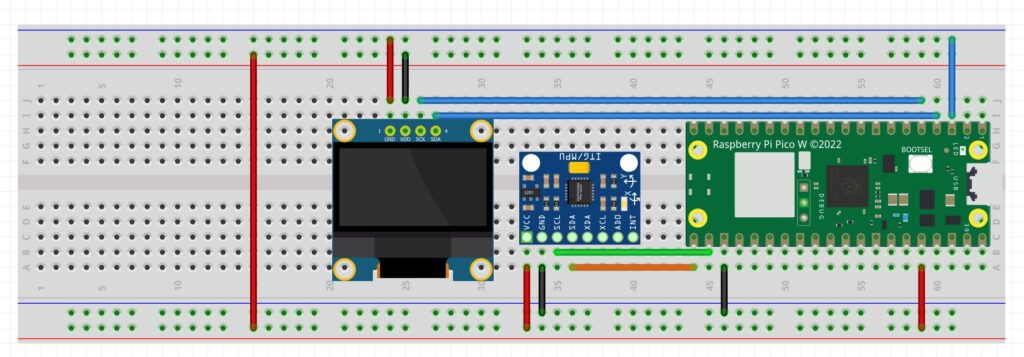
And we also include the code we developed in this lesson.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 | from imu import MPU6050 from machine import I2C,Pin import math import time from ssd1306 import SSD1306_I2C def circle(radius,xOff,yOff): xCenter=64 yCenter=45 for deg in range(0,360): rads=deg/360*2*math.pi x=radius*math.cos(rads) y=radius*math.sin(rads) dsp.pixel(int(x+xCenter-xOff),int(y+yCenter-yOff),1) i2c=I2C(0, sda=Pin(16), scl=Pin(17), freq=400000) mpu = MPU6050(i2c) i2c2=I2C(1,sda=Pin(2), scl=Pin(3), freq=400000) dsp=SSD1306_I2C(128,64,i2c2) dsp.fill(0) while True: xAccel=mpu.accel.x yAccel=mpu.accel.y zAccel=mpu.accel.z pitch=math.atan(yAccel/zAccel) roll=math.atan(xAccel/zAccel) pitchDeg=pitch/(2*math.pi)*360 rollDeg=roll/(2*math.pi)*360 #print('pitch: ',pitchDeg, 'roll: ',rollDeg) dsp.text('Tiltomatic',0,0) msg='P: '+str(round(pitchDeg,1))+' R: '+str(round(rollDeg,1)) dsp.text(msg,0,16) dsp.rect(0,26,128,38,1) dsp.hline(0,45,128,1) dsp.vline(64,26,38,1) print(pitchDeg,rollDeg) circle(7,int(2.5*pitchDeg),int(rollDeg)) dsp.show() dsp.fill(0) time.sleep(.1) |