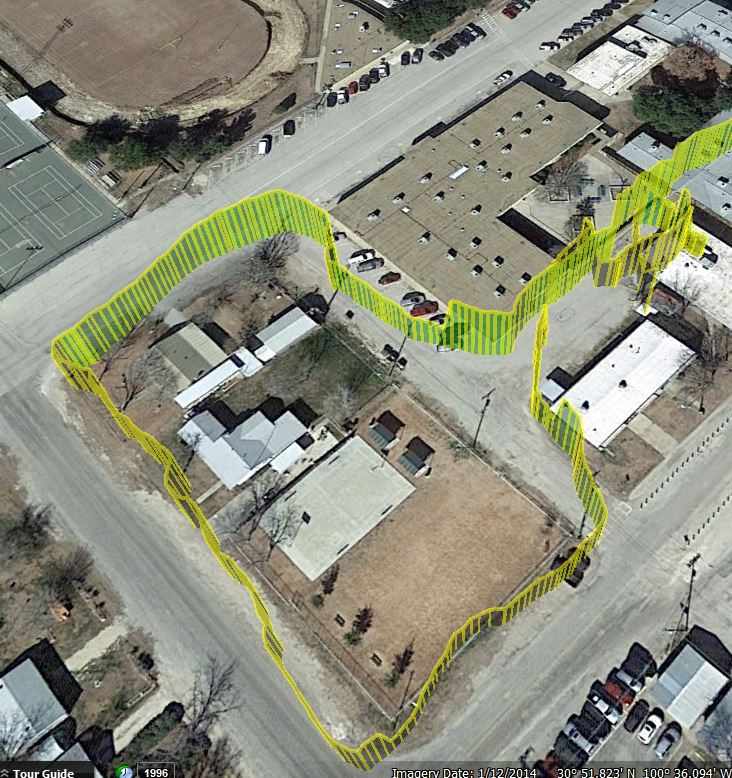
In the earlier lessons in this series we learned how to hook the Adafruit Ultimate GPS up to the Beaglebone Black. In these lessons we got the hardware working, got to the point we could take data, and then got the NMEA sentences parsed, so that we could display understandable data for Latitude, Longitude, and Altitude. In this lesson we will show you how to create a GPS tracker, by logging your GPS data to a file on the Beaglebone Black. Then when you get back home, you can load the data into google earth to see an interactive view of where you have been.
The video shows step-by-step how to get the code working, starting with the code we developed in Lesson 3. We end up with the following program:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 | import serial import Adafruit_BBIO.UART as UART from time import sleep UART.setup("UART1") ser=serial.Serial('/dev/ttyO1',9600) class GPS: def __init__(self): #This sets up variables for useful commands. #This set is used to set the rate the GPS reports UPDATE_10_sec= "$PMTK220,10000*2F\r\n" #Update Every 10 Seconds UPDATE_5_sec= "$PMTK220,5000*1B\r\n" #Update Every 5 Seconds UPDATE_1_sec= "$PMTK220,1000*1F\r\n" #Update Every One Second UPDATE_200_msec= "$PMTK220,200*2C\r\n" #Update Every 200 Milliseconds #This set is used to set the rate the GPS takes measurements MEAS_10_sec = "$PMTK300,10000,0,0,0,0*2C\r\n" #Measure every 10 seconds MEAS_5_sec = "$PMTK300,5000,0,0,0,0*18\r\n" #Measure every 5 seconds MEAS_1_sec = "$PMTK300,1000,0,0,0,0*1C\r\n" #Measure once a second MEAS_200_msec= "$PMTK300,200,0,0,0,0*2F\r\n" #Meaure 5 times a second #Set the Baud Rate of GPS BAUD_57600 = "$PMTK251,57600*2C\r\n" #Set Baud Rate at 57600 BAUD_9600 ="$PMTK251,9600*17\r\n" #Set 9600 Baud Rate #Commands for which NMEA Sentences are sent ser.write(BAUD_57600) sleep(1) ser.baudrate=57600 GPRMC_ONLY= "$PMTK314,0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0*29\r\n" #Send only the GPRMC Sentence GPRMC_GPGGA="$PMTK314,0,1,0,1,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0*28\r\n"#Send GPRMC AND GPGGA Sentences SEND_ALL ="$PMTK314,1,1,1,1,1,1,0,0,0,0,0,0,0,0,0,0,0,0,0*28\r\n" #Send All Sentences SEND_NOTHING="$PMTK314,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0,0*28\r\n" #Send Nothing ser.write(UPDATE_1_sec) sleep(1) ser.write(MEAS_1_sec) sleep(1) ser.write(GPRMC_GPGGA) sleep(1) ser.flushInput() ser.flushInput() print "GPS Initialized" def read(self): ser.flushInput() ser.flushInput() while ser.inWaiting()==0: pass self.NMEA1=ser.readline() while ser.inWaiting()==0: pass self.NMEA2=ser.readline() NMEA1_array=self.NMEA1.split(',') NMEA2_array=self.NMEA2.split(',') if NMEA1_array[0]=='$GPRMC': self.timeUTC=NMEA1_array[1][:-8]+':'+NMEA1_array[1][-8:-6]+':'+NMEA1_array[1][-6:-4] self.latDeg=NMEA1_array[3][:-7] self.latMin=NMEA1_array[3][-7:] self.latHem=NMEA1_array[4] self.lonDeg=NMEA1_array[5][:-7] self.lonMin=NMEA1_array[5][-7:] self.lonHem=NMEA1_array[6] self.knots=NMEA1_array[7] if NMEA1_array[0]=='$GPGGA': self.fix=NMEA1_array[6] self.altitude=NMEA1_array[9] self.sats=NMEA1_array[7] if NMEA2_array[0]=='$GPRMC': self.timeUTC=NMEA2_array[1][:-8]+':'+NMEA1_array[1][-8:-6]+':'+NMEA1_array[1][-6:-4] self.latDeg=NMEA2_array[3][:-7] self.latMin=NMEA2_array[3][-7:] self.latHem=NMEA2_array[4] self.lonDeg=NMEA2_array[5][:-7] self.lonMin=NMEA2_array[5][-7:] self.lonHem=NMEA2_array[6] self.knots=NMEA2_array[7] if NMEA2_array[0]=='$GPGGA': self.fix=NMEA2_array[6] self.altitude=NMEA2_array[9] self.sats=NMEA2_array[7] myGPS=GPS() GPSdata=open('/root/GPS_data/GPS.txt', 'w') GPSdata.close() while(1): myGPS.read() if myGPS.fix!=0: try: latDec=float(myGPS.latDeg)+float(myGPS.latMin)/60. lonDec=float(myGPS.lonDeg)+float(myGPS.lonMin)/60. if myGPS.lonHem=='W': lonDec=(-1)*lonDec if myGPS.latHem=='S': latDec=(-1)*latDec alt=myGPS.altitude GPSdata=open('/root/GPS_data/GPS.txt', 'a') myString=str(lonDec)+','+str(latDec)+','+alt+' ' GPSdata.write(myString) GPSdata.close() except: pass |
This program should create a file on the Beaglebone Black, and track your longitude, latitude and altitude.
In order to view the file on Google Earth, you will want to put a KMZ wrapper on the coordinates. A reasonable KMZ wrapper is below. Simply take your coordinates from the program above, and paste them in the file below, in the area between <coordinates> and </coordinates>
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | <?xml version="1.0" encoding="UTF-8"?> <kml xmlns="http://www.opengis.net/kml/2.2"> <Document> <Style id="yellowPoly"> <LineStyle> <color>7f00ffff</color> <width>4</width> </LineStyle> <PolyStyle> <color>7f00ff00</color> </PolyStyle> </Style> <Placemark><styleUrl>#yellowPoly</styleUrl> <LineString> <extrude>1</extrude> <tesselate>1</tesselate> <altitudeMode>absolute</altitudeMode> <coordinates> </coordinates> </LineString></Placemark> </Document></kml> |